ในการเขียนโปรแกรม MQL4 เราจำเป็นต้องใช้ตัวแปรเพื่อเก็บข้อมูลต่างๆ ตัวแปรเหมือนกับกล่องที่เราใช้เก็บของ โดยแต่ละกล่องจะเก็บของได้เฉพาะประเภท ต่อไปนี้คือประเภทข้อมูลพื้นฐานใน MQL4 และวิธีการใช้งาน:
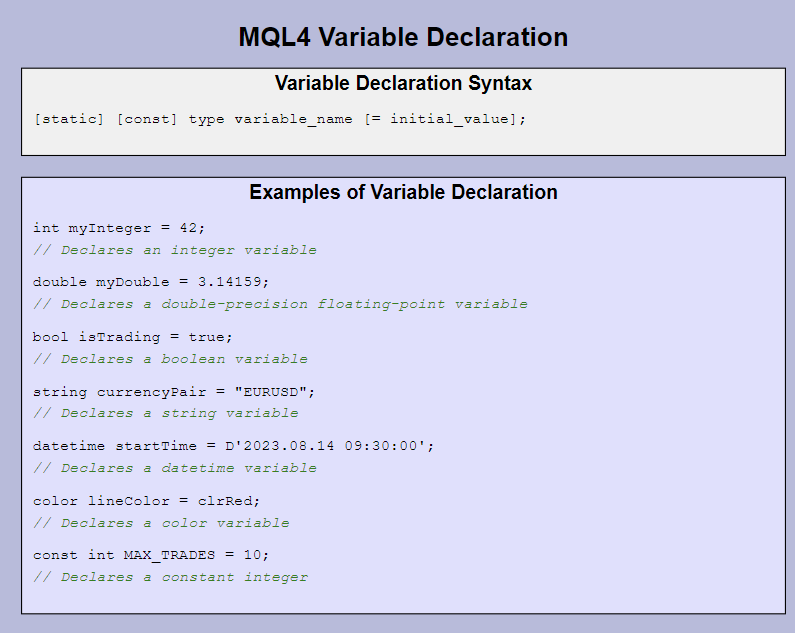
1. ตัวเลขจำนวนเต็ม (Integer)
ใช้เก็บตัวเลขที่ไม่มีจุดทศนิยม
int myAge = 25;
int lotSize = 1;
int orderNumber = 12345;
Print("My age is ", myAge);
Print("Lot size is ", lotSize);
Print("Order number is ", orderNumber);
2. ตัวเลขทศนิยม (Double)
ใช้เก็บตัวเลขที่มีจุดทศนิยม เหมาะสำหรับราคาหรือค่าที่ต้องการความแม่นยำสูง
double currentPrice = 1.2345;
double stopLoss = 1.2300;
double takeProfit = 1.2400;
Print("Current price is ", currentPrice);
Print("Stop Loss is set at ", stopLoss);
Print("Take Profit is set at ", takeProfit);
3. ข้อความ (String)
ใช้เก็บตัวอักษรหรือข้อความ
string myName = "John Doe";
string currencyPair = "EURUSD";
string tradeComment = "My first trade";
Print("My name is ", myName);
Print("Trading on ", currencyPair);
Print("Trade comment: ", tradeComment);
4. ค่าความจริง (Boolean)
ใช้เก็บค่าจริง (true) หรือเท็จ (false)
bool isMarketOpen = true;
bool hasOpenTrades = false;
if(isMarketOpen) {
Print("The market is open");
}
if(!hasOpenTrades) {
Print("There are no open trades");
}
5. วันที่และเวลา (Datetime)
ใช้เก็บข้อมูลวันที่และเวลา
datetime currentTime = TimeCurrent();
datetime orderOpenTime = D'2023.05.01 09:00';
Print("Current time is ", TimeToString(currentTime));
Print("Order was opened at ", TimeToString(orderOpenTime));
6. สี (Color)
ใช้กำหนดสีสำหรับกราฟหรือIndicator
color buyColor = clrGreen;
color sellColor = clrRed;
color neutralColor = clrGray;
Print("Buy color is ", ColorToString(buyColor));
Print("Sell color is ", ColorToString(sellColor));
Print("Neutral color is ", ColorToString(neutralColor));
7. อาร์เรย์ (Array)
ใช้เก็บข้อมูลหลายๆ ค่าในตัวแปรเดียว
double prices[5] = {1.2345, 1.2346, 1.2347, 1.2348, 1.2349};
string days[] = {"Monday", "Tuesday", "Wednesday", "Thursday", "Friday"};
Print("Third price in the array is ", prices[2]);
Print("The fourth day is ", days[3]);
การใช้งานตัวแปรในสถานการณ์จริง
ต่อไปนี้เป็นตัวอย่างการใช้ตัวแปรต่างๆ ในการสร้างคำสั่งซื้อขาย:
void PlaceOrder()
{
string symbol = "EURUSD";
int orderType = OP_BUY;
double lotSize = 0.1;
double entryPrice = Ask;
double stopLoss = Ask - 50 * Point;
double takeProfit = Ask + 100 * Point;
string comment = "My EA Trade";
color arrowColor = clrBlue;
int ticket = OrderSend(symbol, orderType, lotSize, entryPrice, 3, stopLoss, takeProfit, comment, 0, 0, arrowColor);
if(ticket > 0) {
Print("Order placed successfully. Ticket: ", ticket);
} else {
Print("Error placing order. Error code: ", GetLastError());
}
}
ในตัวอย่างนี้ เราใช้ตัวแปรหลายประเภทเพื่อกำหนดพารามิเตอร์ต่างๆ สำหรับการเปิดคำสั่งซื้อขาย
การเข้าใจวิธีการประกาศและใช้งานตัวแปรประเภทต่างๆ เป็นพื้นฐานสำคัญในการเขียนโปรแกรม EA ที่มีประสิทธิภาพ เมื่อคุณคุ้นเคยกับการใช้ตัวแปรเหล่านี้ คุณจะสามารถสร้างโลจิกการเทรดที่ซับซ้อนและยืดหยุ่นมากขึ้นได้
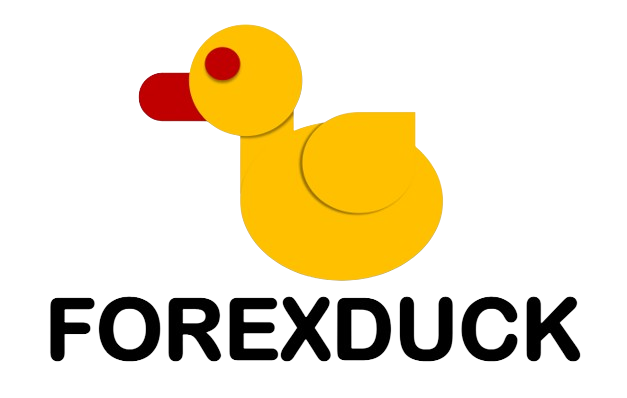
FOREXDUCK (นามปากกา) นักเขียนของเรามีประสบการณ์การเงินการลงทุนกว่า 10 ปี มีความเชี่ยวชาญในการวิเคราะห์ตลาด Forex และคริปโต โดยเฉพาะการวิเคราะห์ทางเทคนิค รวมถึงเทคนิคต่าง