ตัวอย่าง EA ที่ใช้หลายกลยุทธ์ร่วมกัน
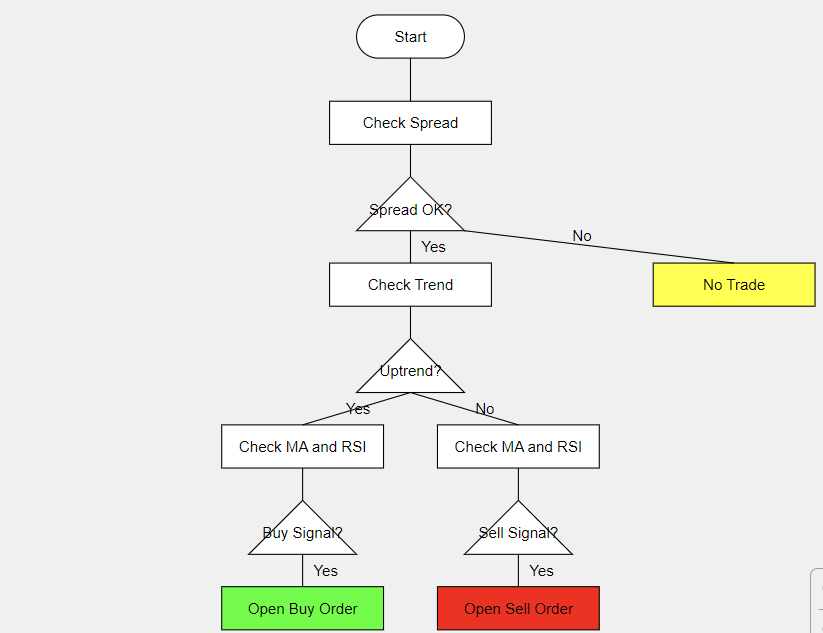
คำอธิบายองค์ประกอบหลักของ EA ที่ใช้หลายกลยุทธ์ร่วมกัน:
- กลยุทธ์ที่ใช้:
- Trend Following: ใช้ Trend MA เพื่อกำหนดแนวโน้มหลัก
- MA Crossover: ใช้การตัดกันของ Fast MA และ Slow MA
- RSI: ใช้ RSI เพื่อระบุภาวะ overbought และ oversold
- การตัดสินใจเข้าเทรด:
- Buy: เมื่อราคาอยู่เหนือ Trend MA และ (Fast MA ตัดขึ้นบน Slow MA หรือ RSI < oversold)
- Sell: เมื่อราคาอยู่ใต้ Trend MA และ (Fast MA ตัดลงใต้ Slow MA หรือ RSI > overbought)
- การจัดการความเสี่ยง:
- ใช้ Stop Loss และ Take Profit
- ใช้ Trailing Stop เพื่อปรับ Stop Loss ตามราคาที่เคลื่อนไหว
- การออกจากเทรด:
- ปิดเทรดเมื่อมีสัญญาณตรงข้ามเกิดขึ้น
//+------------------------------------------------------------------+
//| Multi-Strategy EA.mq4 |
//| Copyright 2024, MetaQuotes Software Corp. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2024, MetaQuotes Software Corp."
#property link "https://www.mql5.com"
#property version "1.00"
#property strict
// Input parameters
input int TrendMA = 200; // Trend MA period
input int FastMA = 10; // Fast MA period
input int SlowMA = 30; // Slow MA period
input int RSI_Period = 14; // RSI period
input int RSI_Overbought = 70; // RSI overbought level
input int RSI_Oversold = 30; // RSI oversold level
input double LotSize = 0.01; // Lot size for each trade
input int StopLoss = 50; // Stop Loss in pips
input int TakeProfit = 100; // Take Profit in pips
input int MaxSpread = 5; // Maximum allowed spread in pips
input int MagicNumber = 12345; // Magic number for order identification
// Global variables
double g_pip;
int g_trendMA_handle, g_fastMA_handle, g_slowMA_handle, g_rsi_handle;
double g_trendMA_buffer[], g_fastMA_buffer[], g_slowMA_buffer[], g_rsi_buffer[];
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
// Initialize pip value
g_pip = Point * 10;
// Initialize indicators
g_trendMA_handle = iMA(NULL, 0, TrendMA, 0, MODE_SMA, PRICE_CLOSE);
g_fastMA_handle = iMA(NULL, 0, FastMA, 0, MODE_SMA, PRICE_CLOSE);
g_slowMA_handle = iMA(NULL, 0, SlowMA, 0, MODE_SMA, PRICE_CLOSE);
g_rsi_handle = iRSI(NULL, 0, RSI_Period, PRICE_CLOSE);
if (g_trendMA_handle == INVALID_HANDLE || g_fastMA_handle == INVALID_HANDLE ||
g_slowMA_handle == INVALID_HANDLE || g_rsi_handle == INVALID_HANDLE)
{
Print("Error initializing indicators");
return INIT_FAILED;
}
// Allocate memory for indicator buffers
ArraySetAsSeries(g_trendMA_buffer, true);
ArraySetAsSeries(g_fastMA_buffer, true);
ArraySetAsSeries(g_slowMA_buffer, true);
ArraySetAsSeries(g_rsi_buffer, true);
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
// Release indicator handles
IndicatorRelease(g_trendMA_handle);
IndicatorRelease(g_fastMA_handle);
IndicatorRelease(g_slowMA_handle);
IndicatorRelease(g_rsi_handle);
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
// Check if spread is too high
if (MarketInfo(Symbol(), MODE_SPREAD) > MaxSpread)
{
return;
}
// Update indicator values
if (CopyBuffer(g_trendMA_handle, 0, 0, 2, g_trendMA_buffer) <= 0) return;
if (CopyBuffer(g_fastMA_handle, 0, 0, 2, g_fastMA_buffer) <= 0) return;
if (CopyBuffer(g_slowMA_handle, 0, 0, 2, g_slowMA_buffer) <= 0) return;
if (CopyBuffer(g_rsi_handle, 0, 0, 2, g_rsi_buffer) <= 0) return; // Check for entry signals if (!IsTradeOpen()) { if (IsBuySignal()) { OpenBuyTrade(); } else if (IsSellSignal()) { OpenSellTrade(); } } // Manage open trades ManageOpenTrades(); } //+------------------------------------------------------------------+ //| Check for buy signal | //+------------------------------------------------------------------+ bool IsBuySignal() { // Trend following bool uptrend = Close[0] > g_trendMA_buffer[0];
// MA crossover
bool ma_crossover = g_fastMA_buffer[1] <= g_slowMA_buffer[1] && g_fastMA_buffer[0] > g_slowMA_buffer[0];
// RSI oversold
bool rsi_oversold = g_rsi_buffer[0] < RSI_Oversold;
return uptrend && (ma_crossover || rsi_oversold);
}
//+------------------------------------------------------------------+
//| Check for sell signal |
//+------------------------------------------------------------------+
bool IsSellSignal()
{
// Trend following
bool downtrend = Close[0] < g_trendMA_buffer[0]; // MA crossover bool ma_crossover = g_fastMA_buffer[1] >= g_slowMA_buffer[1] && g_fastMA_buffer[0] < g_slowMA_buffer[0]; // RSI overbought bool rsi_overbought = g_rsi_buffer[0] > RSI_Overbought;
return downtrend && (ma_crossover || rsi_overbought);
}
//+------------------------------------------------------------------+
//| Check if there's an open trade |
//+------------------------------------------------------------------+
bool IsTradeOpen()
{
for (int i = 0; i < OrdersTotal(); i++) { if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES)) { if (OrderSymbol() == Symbol() && OrderMagicNumber() == MagicNumber) { return true; } } } return false; } //+------------------------------------------------------------------+ //| Open a buy trade | //+------------------------------------------------------------------+ void OpenBuyTrade() { double sl = Ask - StopLoss * g_pip; double tp = Ask + TakeProfit * g_pip; int ticket = OrderSend(Symbol(), OP_BUY, LotSize, Ask, 3, sl, tp, "Multi-Strategy Buy", MagicNumber, 0, Blue); if (ticket > 0)
{
Print("Buy order opened: ", ticket);
}
else
{
Print("Error opening buy order: ", GetLastError());
}
}
//+------------------------------------------------------------------+
//| Open a sell trade |
//+------------------------------------------------------------------+
void OpenSellTrade()
{
double sl = Bid + StopLoss * g_pip;
double tp = Bid - TakeProfit * g_pip;
int ticket = OrderSend(Symbol(), OP_SELL, LotSize, Bid, 3, sl, tp, "Multi-Strategy Sell", MagicNumber, 0, Red);
if (ticket > 0)
{
Print("Sell order opened: ", ticket);
}
else
{
Print("Error opening sell order: ", GetLastError());
}
}
//+------------------------------------------------------------------+
//| Manage open trades |
//+------------------------------------------------------------------+
void ManageOpenTrades()
{
for (int i = 0; i < OrdersTotal(); i++) { if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES)) { if (OrderSymbol() == Symbol() && OrderMagicNumber() == MagicNumber) { // Check for exit signals if (OrderType() == OP_BUY && IsSellSignal()) { CloseTrade(); } else if (OrderType() == OP_SELL && IsBuySignal()) { CloseTrade(); } // Implement trailing stop TrailingStop(30); } } } } //+------------------------------------------------------------------+ //| Close the current trade | //+------------------------------------------------------------------+ void CloseTrade() { if (OrderType() == OP_BUY) { if (!OrderClose(OrderTicket(), OrderLots(), Bid, 3, Blue)) { Print("Error closing buy order: ", GetLastError()); } } else if (OrderType() == OP_SELL) { if (!OrderClose(OrderTicket(), OrderLots(), Ask, 3, Red)) { Print("Error closing sell order: ", GetLastError()); } } } //+------------------------------------------------------------------+ //| Implement trailing stop | //+------------------------------------------------------------------+ void TrailingStop(int pips) { double newSL; if (OrderType() == OP_BUY) { newSL = Bid - pips * g_pip; if (newSL > OrderStopLoss() && newSL < Bid)
{
if (!OrderModify(OrderTicket(), OrderOpenPrice(), newSL, OrderTakeProfit(), 0, Blue))
{
Print("Error modifying buy order: ", GetLastError());
}
}
}
else if (OrderType() == OP_SELL)
{
newSL = Ask + pips * g_pip;
if ((newSL < OrderStopLoss() || OrderStopLoss() == 0) && newSL > Ask)
{
if (!OrderModify(OrderTicket(), OrderOpenPrice(), newSL, OrderTakeProfit(), 0, Red))
{
Print("Error modifying sell order: ", GetLastError());
}
}
}
}
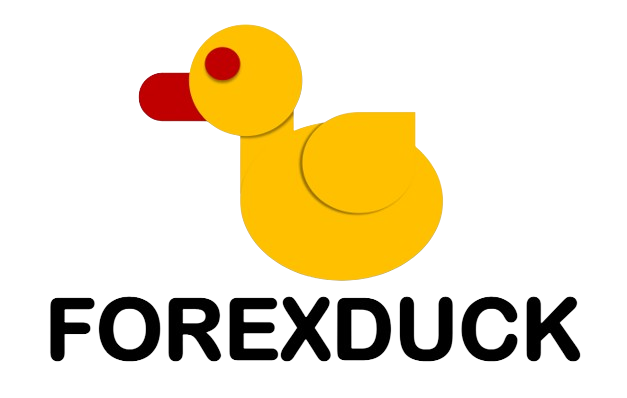
FOREXDUCK (นามปากกา) นักเขียนของเรามีประสบการณ์การเงินการลงทุนกว่า 10 ปี มีความเชี่ยวชาญในการวิเคราะห์ตลาด Forex และคริปโต โดยเฉพาะการวิเคราะห์ทางเทคนิค รวมถึงเทคนิคต่าง