ตัวอย่าง EA แบบ Scalping
คำอธิบายองค์ประกอบหลักของ EA แบบ Scalping:
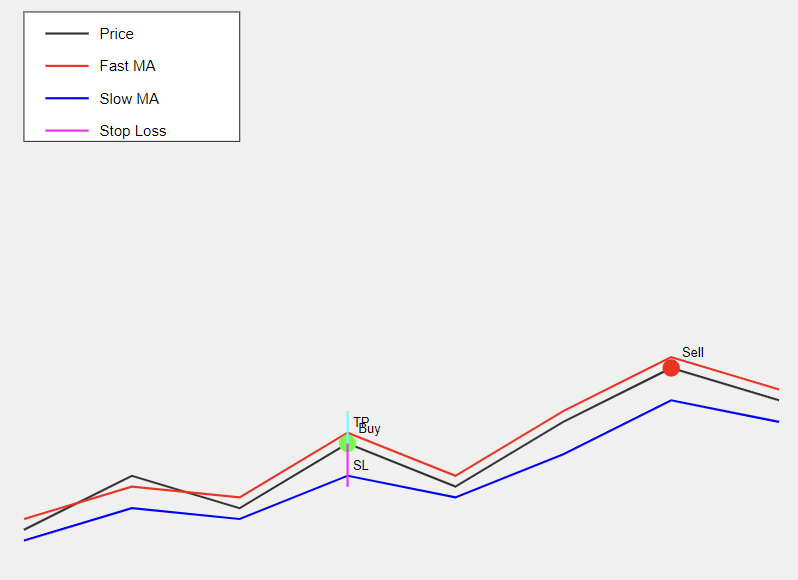
- Input Parameters:
- FastMA และ SlowMA: ช่วงเวลาสำหรับ Moving Average เร็วและช้า
- LotSize: ขนาดล็อตสำหรับแต่ละการเทรด
- StopLoss และ TakeProfit: ระยะ Stop Loss และ Take Profit ในหน่วย pips
- MaxSpread: ค่า spread สูงสุดที่ยอมรับได้
- Slippage: ค่า slippage สูงสุดที่ยอมรับได้
- OnInit():
- สร้างและตั้งค่า Moving Average indicators
- OnTick():
- ตรวจสอบ spread
- อัพเดทค่า indicators
- ตรวจสอบสัญญาณเข้าและออกจากตลาด
- IsTradeOpen():
- ตรวจสอบว่ามีการเทรดที่เปิดอยู่หรือไม่
- OpenBuyTrade() และ OpenSellTrade():
- เปิดออเดอร์ Buy หรือ Sell พร้อมกำหนด Stop Loss และ Take Profit
- ManageOpenTrades():
- จัดการออเดอร์ที่เปิดอยู่ ตรวจสอบสัญญาณออกจากตลาด และปรับ trailing stop
- CloseTrade():
- ปิดออเดอร์ที่เปิดอยู่
- TrailingStop():
- ปรับ Stop Loss แบบ trailing stop
EA นี้ใช้การตัดกันของ Moving Average สองเส้นเพื่อสร้างสัญญาณการเทรด โดยจะเปิด Buy เมื่อ Fast MA ตัดขึ้นบน Slow MA และเปิด Sell เมื่อ Fast MA ตัดลงใต้ Slow MA นอกจากนี้ยังมีการจัดการความเสี่ยงด้วยการกำหนด Stop Loss, Take Profit และใช้ trailing stop
//+------------------------------------------------------------------+
//| Scalping EA.mq4 |
//| Copyright 2024, MetaQuotes Software Corp. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2024, MetaQuotes Software Corp."
#property link "https://www.mql5.com"
#property version "1.00"
#property strict
// Input parameters
input int FastMA = 10; // Fast Moving Average period
input int SlowMA = 30; // Slow Moving Average period
input double LotSize = 0.01; // Lot size for each trade
input int StopLoss = 20; // Stop Loss in pips
input int TakeProfit = 10; // Take Profit in pips
input int MaxSpread = 3; // Maximum allowed spread in pips
input int Slippage = 3; // Maximum allowed slippage in pips
input int MagicNumber = 12345; // Magic number for order identification
// Global variables
double g_pip;
int g_fastMA_handle, g_slowMA_handle;
double g_fastMA_buffer[], g_slowMA_buffer[];
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
// Initialize pip value
g_pip = Point * 10;
// Initialize MA indicators
g_fastMA_handle = iMA(NULL, 0, FastMA, 0, MODE_SMA, PRICE_CLOSE);
g_slowMA_handle = iMA(NULL, 0, SlowMA, 0, MODE_SMA, PRICE_CLOSE);
if (g_fastMA_handle == INVALID_HANDLE || g_slowMA_handle == INVALID_HANDLE)
{
Print("Error initializing MA indicators");
return INIT_FAILED;
}
// Allocate memory for indicator buffers
ArraySetAsSeries(g_fastMA_buffer, true);
ArraySetAsSeries(g_slowMA_buffer, true);
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
// Release indicator handles
IndicatorRelease(g_fastMA_handle);
IndicatorRelease(g_slowMA_handle);
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
// Check if spread is too high
if (MarketInfo(Symbol(), MODE_SPREAD) > MaxSpread)
{
return;
}
// Update indicator values
if (CopyBuffer(g_fastMA_handle, 0, 0, 2, g_fastMA_buffer) <= 0) return;
if (CopyBuffer(g_slowMA_handle, 0, 0, 2, g_slowMA_buffer) <= 0) return;
// Check for entry signals
if (g_fastMA_buffer[1] <= g_slowMA_buffer[1] && g_fastMA_buffer[0] > g_slowMA_buffer[0])
{
if (!IsTradeOpen())
{
OpenBuyTrade();
}
}
else if (g_fastMA_buffer[1] >= g_slowMA_buffer[1] && g_fastMA_buffer[0] < g_slowMA_buffer[0])
{
if (!IsTradeOpen())
{
OpenSellTrade();
}
}
// Check for exit signals
ManageOpenTrades();
}
//+------------------------------------------------------------------+
//| Check if there's an open trade |
//+------------------------------------------------------------------+
bool IsTradeOpen()
{
for (int i = 0; i < OrdersTotal(); i++) { if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES)) { if (OrderSymbol() == Symbol() && OrderMagicNumber() == MagicNumber) { return true; } } } return false; } //+------------------------------------------------------------------+ //| Open a buy trade | //+------------------------------------------------------------------+ void OpenBuyTrade() { double sl = Ask - StopLoss * g_pip; double tp = Ask + TakeProfit * g_pip; int ticket = OrderSend(Symbol(), OP_BUY, LotSize, Ask, Slippage, sl, tp, "Scalping Buy", MagicNumber, 0, Blue); if (ticket > 0)
{
Print("Buy order opened: ", ticket);
}
else
{
Print("Error opening buy order: ", GetLastError());
}
}
//+------------------------------------------------------------------+
//| Open a sell trade |
//+------------------------------------------------------------------+
void OpenSellTrade()
{
double sl = Bid + StopLoss * g_pip;
double tp = Bid - TakeProfit * g_pip;
int ticket = OrderSend(Symbol(), OP_SELL, LotSize, Bid, Slippage, sl, tp, "Scalping Sell", MagicNumber, 0, Red);
if (ticket > 0)
{
Print("Sell order opened: ", ticket);
}
else
{
Print("Error opening sell order: ", GetLastError());
}
}
//+------------------------------------------------------------------+
//| Manage open trades |
//+------------------------------------------------------------------+
void ManageOpenTrades()
{
for (int i = 0; i < OrdersTotal(); i++)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if (OrderSymbol() == Symbol() && OrderMagicNumber() == MagicNumber)
{
// Check for exit signals
if (OrderType() == OP_BUY && g_fastMA_buffer[0] < g_slowMA_buffer[0]) { CloseTrade(); } else if (OrderType() == OP_SELL && g_fastMA_buffer[0] > g_slowMA_buffer[0])
{
CloseTrade();
}
// Implement trailing stop
TrailingStop(20);
}
}
}
}
//+------------------------------------------------------------------+
//| Close the current trade |
//+------------------------------------------------------------------+
void CloseTrade()
{
if (OrderType() == OP_BUY)
{
if (!OrderClose(OrderTicket(), OrderLots(), Bid, Slippage, Blue))
{
Print("Error closing buy order: ", GetLastError());
}
}
else if (OrderType() == OP_SELL)
{
if (!OrderClose(OrderTicket(), OrderLots(), Ask, Slippage, Red))
{
Print("Error closing sell order: ", GetLastError());
}
}
}
//+------------------------------------------------------------------+
//| Implement trailing stop |
//+------------------------------------------------------------------+
void TrailingStop(int pips)
{
double newSL;
if (OrderType() == OP_BUY)
{
newSL = Bid - pips * g_pip;
if (newSL > OrderStopLoss() && newSL < Bid)
{
if (!OrderModify(OrderTicket(), OrderOpenPrice(), newSL, OrderTakeProfit(), 0, Blue))
{
Print("Error modifying buy order: ", GetLastError());
}
}
}
else if (OrderType() == OP_SELL)
{
newSL = Ask + pips * g_pip;
if ((newSL < OrderStopLoss() || OrderStopLoss() == 0) && newSL > Ask)
{
if (!OrderModify(OrderTicket(), OrderOpenPrice(), newSL, OrderTakeProfit(), 0, Red))
{
Print("Error modifying sell order: ", GetLastError());
}
}
}
}
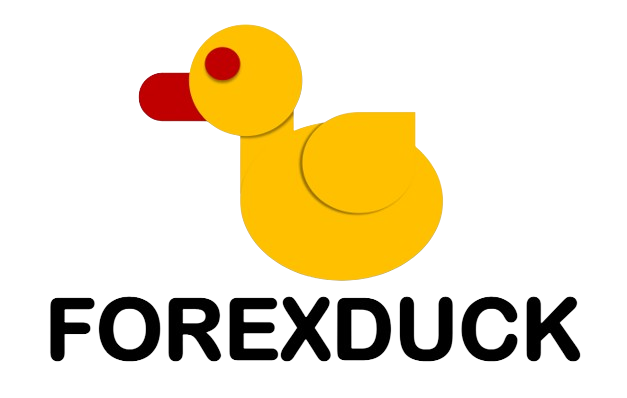
FOREXDUCK (นามปากกา) นักเขียนของเรามีประสบการณ์การเงินการลงทุนกว่า 10 ปี มีความเชี่ยวชาญในการวิเคราะห์ตลาด Forex และคริปโต โดยเฉพาะการวิเคราะห์ทางเทคนิค รวมถึงเทคนิคต่าง